- Home
- Engineering
- What are the Best Practices fo ...
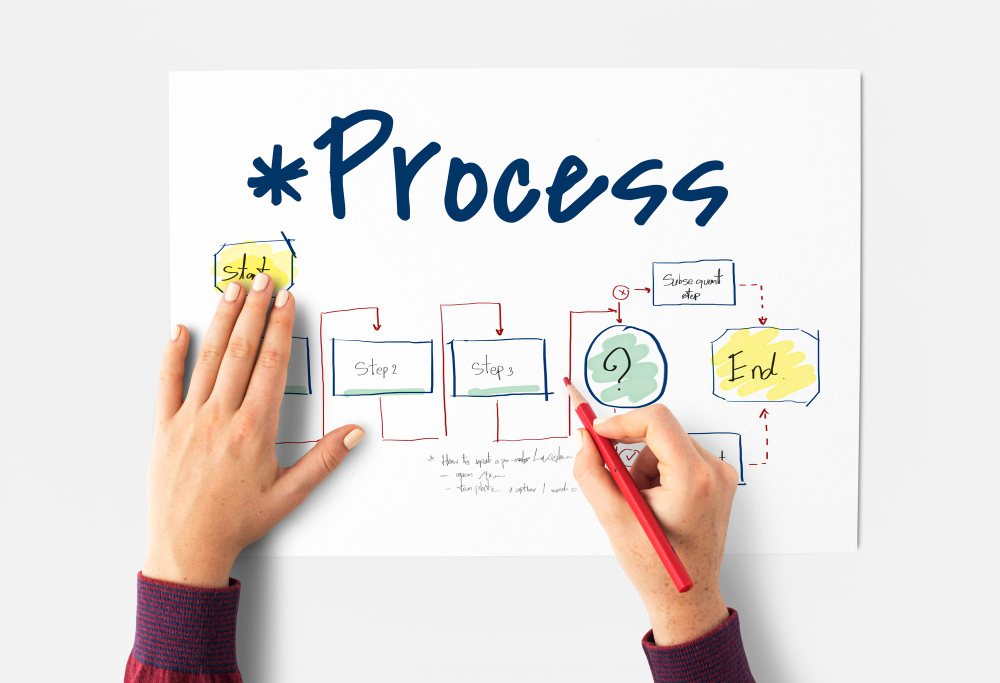
Imagine you’re running a busy online store. When a customer orders, you want to send them a confirmation email and process their payment immediately. But these tasks can take time, and you don’t want to slow down your website for other customers.
This is where Laravel background jobs come in. They allow you to offload these time-consuming tasks to run in the background, without affecting the performance of your application. This way, your website can continue to serve other customers quickly while the background jobs take care of the order confirmation and payment processing.
Why use background jobs?
- Improved Performance: By moving time-consuming tasks to the background, you can significantly improve the responsiveness of your application.
- Enhanced User Experience: Faster response times and smoother interactions lead to a better user experience.
- Scalability: Background jobs can help your application scale by distributing the workload across multiple workers.
- Reliability: Even if a background job fails, you can retry it or handle the error gracefully.
In the next sections, we’ll dive deeper into how to implement and optimize background jobs in your Laravel applications.
Understanding Laravel’s Queue System: A Simple Analogy
Imagine a busy restaurant kitchen. The chefs (queue workers) are responsible for preparing the food (queue jobs). They get their orders from a queue (queue driver), which can be a whiteboard, a digital tablet, or a voice command system.
In Laravel, it’s similar:
- Queue Workers: These are like the chefs. They continuously check the queue for new jobs and process them one by one.
- Queue Drivers: This is the system used to store the queue of jobs. Laravel supports various drivers, such as:
- Database: Stores jobs in a database table.
- Redis: A fast in-memory data store.
- Beanstalkd: A high-performance workqueue system.
- Amazon SQS: A scalable and reliable message queue service.
- Queue Jobs: These are the individual tasks that need to be processed, like cooking a specific dish. They can be simple tasks like sending an email or complex tasks like processing a large data file.
By using Laravel’s queue system, you can offload these tasks to background workers, allowing your application to respond quickly to user requests.
Choosing the Right Queue Driver
Let’s dive deeper into the different queue drivers available in Laravel and when to use them.
Database Driver
- How it works: Stores jobs in a database table.
- Best for:
- Simple applications: If you have a small-scale application and don’t require high performance or reliability, the database driver is a good starting point.
- Local development: It’s easy to set up and debug.
Redis Driver
- How it works: Uses Redis, an in-memory data store, to store jobs.
- Best for:
- Medium to large-scale applications: Redis offers excellent performance and reliability.
- Real-time processing: It’s ideal for jobs that need to be processed immediately.
Beanstalkd Driver
- How it works: Uses Beanstalkd, a high-performance workqueue system, to store jobs.
- Best for:
- High-performance applications: Beanstalkd is optimized for performance and reliability.
- Complex job queues: It supports features like priorities, time-to-live, and delay.
Amazon SQS Driver
- How it works: Uses Amazon Simple Queue Service (SQS) to store jobs.
- Best for:
- Large-scale, distributed applications: SQS is a highly scalable and durable message queue service.
- Cloud-based applications: It’s a good choice if you’re using AWS for your infrastructure.
Choosing the right driver:
- Performance: Consider the performance requirements of your application. Redis and Beanstalkd are generally faster than the database driver.
- Reliability: If reliability is a top priority, Amazon SQS is a good option.
- Scalability: For large-scale applications, Redis and Amazon SQS are more scalable than the database driver.
- Cost: Consider the cost of using a cloud-based service like Amazon SQS.
By carefully considering these factors, you can choose the best queue driver for your Laravel application.
Best Practices for Implementing Background Jobs
Choosing the Right Queue Driver
As we’ve discussed, Laravel offers several queue drivers. Let’s delve deeper into the factors to consider when selecting the right one:
Performance:
- Redis and Beanstalkd are known for their high performance, making them ideal for real-time processing and high-traffic applications.
- Database drivers, while simpler, can be slower for large-scale applications.
Reliability:
- Amazon SQS offers robust reliability and durability, making it suitable for mission-critical applications.
- Redis and Beanstalkd are also reliable but may require additional configuration for high availability.
Cost:
- Database and Redis drivers are typically free to use.
- Beanstalkd may require hosting or self-hosting.
- Amazon SQS has associated costs based on the number of messages sent and received.
Designing Efficient Jobs
- Single Responsibility Principle: Each job should focus on a single task. This makes them easier to test, debug, and maintain.
- Minimize Database Interactions: Excessive database queries can slow down job processing. Consider using caching or bulk operations to reduce the number of queries.
- Optimize API Calls: Limit API calls to essential data. Use rate limiting and caching to reduce the load on external services.
- Robust Error Handling: Implement proper error handling and logging to identify and fix issues promptly. Use retry mechanisms for transient errors.
Optimizing Job Processing
- Configure Queue Workers: Adjust the number of workers based on your application’s workload. Too many workers can lead to resource contention, while too few can result in job backlogs.
- Rate Limiting: Prevent your application from being overwhelmed by limiting the rate at which jobs are processed.
- Job Chaining: Execute multiple related jobs sequentially or concurrently to streamline workflows.
Securing Background Jobs
- Data Encryption: Protect sensitive data by encrypting it before storing or transmitting it.
- Secure Communication: Use secure channels (e.g., HTTPS) to communicate with external services.
- Authentication and Authorization: Implement robust authentication and authorization mechanisms to control access to jobs and sensitive data.
Monitoring and Debugging
- Laravel’s Built-in Tools: Utilize Laravel’s built-in tools to monitor queue health, job processing, and failures.
- Custom Logging: Implement custom logging to track specific events and errors.
- Debugging Techniques: Use debugging tools and techniques to identify and fix issues in your jobs.
By following these best practices, you can effectively implement background jobs in your Laravel applications, improving performance, scalability, and reliability.
Using Job Middleware: Enhancing Job Functionality
What is Job Middleware?
Think of job middleware as a set of filters that can be applied to your background jobs. These filters can add additional functionality, such as logging, authentication, or custom error handling.
How to Create Custom Job Middleware
- Create a Middleware Class:
php artisan make:middleware LogJobMiddleware
- Implement the Handle Method:
namespace App\Http\Middleware;
use Closure;
class LogJobMiddleware
{
public function handle($job, Closure $next)
{
// Log the job's information
Log::info('Job started: ' . $job->jobName());
// Continue to the next middleware or the job's handle method
$result = $next($job);
// Log the job's completion
Log::info('Job finished: ' . $job->jobName());
return $result;
}
}
Register the Middleware: In app/Http/Kernel.php
, add the middleware to the $middleware
array:
protected $middleware = [
// ... other middleware
App\Http\Middleware\LogJobMiddleware::class,
];
Applying Middleware to Jobs
To apply the middleware to a specific job, use the withMiddleware
method on the job instance:
MyJob::dispatch()->withMiddleware(LogJobMiddleware::class);
Common Use Cases for Job Middleware:
- Logging: Log job start, finish, and error events.
- Authentication: Verify user credentials before executing a job.
- Authorization: Check if the user is authorized to perform the job’s actions.
- Error Handling: Implement custom error handling and notifications.
- Rate Limiting: Limit the number of jobs that can be processed within a certain time frame.
- Caching: Cache job results to improve performance.
By using job middleware, you can easily add powerful functionality to your background jobs, making them more robust, secure, and efficient.
Scheduling Jobs with Laravel’s Scheduler
Laravel’s scheduler provides a convenient way to automate tasks and schedule jobs to run at specific times or intervals.
Setting Up the Scheduler
Enable the Scheduler: In your AppServiceProvider
or a custom service provider, add the ScheduleServiceProvider
to the providers
array:
public function register() { $this->app->register(App\Providers\ScheduleServiceProvider::class); }
Define the Schedule: Create a Schedule
class in your app/Console/Kernel.php
file and define your scheduled tasks:
protected function schedule(Schedule $schedule) { $schedule->command('your:command')->dailyAt('13:00'); $schedule->call(function () { // Your task here })->everyMinute(); }
Scheduling Examples
- Running a Command Daily:
$schedule->command('your:command')->daily();
- Running a Command Every Minute:
$schedule->command('your:command')->everyMinute();
- Running a Command Hourly:
$schedule->command('your:command')->hourly();
- Running a Command Weekly:
$schedule->command('your:command')->weekly();
- Running a Command Monthly:
$schedule->command('your:command')->monthly();
- Running a Command at a Specific Time:
$schedule->command('your:command')->dailyAt('13:00');
Running the Scheduler
To run the scheduler, use the following command in your terminal:
php artisan schedule:run
Use code with caution.
Additional Tips
- Using Cron Jobs: For more complex scheduling scenarios or environments without a web server, consider using cron jobs.
- Testing Schedules: Use Laravel’s testing tools to test your scheduled tasks.
- Error Handling: Implement proper error handling and logging for scheduled tasks.
- Environment Variables: Use environment variables to configure dynamic scheduling options.
By effectively utilizing Laravel’s scheduler, you can automate routine tasks, improve efficiency, and streamline your application’s maintenance.
Testing Background Jobs: Ensuring Reliability
Testing background jobs is crucial to ensure their correct functionality and prevent unexpected issues. Here are some tips for effectively testing your Laravel background jobs:
Testing Jobs in Isolation
- Unit Testing:
- Mock Dependencies: Use mocking frameworks like PHPUnit’s Mockery to isolate the job and replace its dependencies with mock objects.
- Test Job Logic: Focus on testing the core logic of the job, such as data processing, API calls, and database interactions.
- Assert Expected Behavior: Use PHPUnit’s assertion methods to verify the job’s output and side effects.
- Functional Testing:
- Simulate Job Execution: Trigger the job and observe its behavior in a controlled environment.
- Inspect Job Results: Check the job’s output, database changes, and any external system interactions.
- Use Test Databases: Use a separate test database to avoid affecting production data.
Testing Jobs Within the Application Context
- Integration Testing:
- Test Job Scheduling: Verify that jobs are scheduled correctly and executed at the right time.
- Test Job Queuing and Processing: Ensure that jobs are added to the queue and processed by workers.
- Test Job Failures and Retries: Simulate job failures and verify that retry mechanisms work as expected.
- End-to-End Testing:
- Test User Interactions: Trigger actions that initiate background jobs and observe the system’s response.
- Verify Job Outcomes: Check the final state of the system after job completion.
- Use Test Data: Use a combination of test data and real-world data to simulate different scenarios.
Additional Tips:
- Use a Test Environment: Set up a dedicated test environment with separate databases, queues, and other resources.
- Leverage Testing Tools: Utilize Laravel’s testing framework and other testing tools to simplify the testing process.
- Write Clear and Concise Tests: Write tests that are easy to understand and maintain.
- Test Edge Cases: Consider edge cases and unexpected input to ensure robust job behavior.
- Monitor Job Performance: Use monitoring tools to track job execution times and identify performance bottlenecks.
By following these guidelines, you can effectively test your background jobs and ensure the reliability of your Laravel applications.
Wrapping Up: Building Robust Background Jobs in Laravel
In this article, we’ve explored the power of Laravel’s background job system and how to implement it effectively.
We’ve covered:
- Understanding the Basics: We’ve broken down the core components of Laravel’s queue system, including queue workers, drivers, and jobs.
- Choosing the Right Queue Driver: We’ve discussed the pros and cons of different drivers like database, Redis, Beanstalkd, and Amazon SQS.
- Designing Efficient Jobs: We’ve emphasized the importance of keeping jobs focused, minimizing database interactions, and implementing robust error handling.
- Optimizing Job Processing: We’ve explored techniques like configuring queue workers, rate limiting, and job chaining to optimize performance.
- Securing Background Jobs: We’ve discussed the importance of protecting sensitive data and implementing authentication and authorization mechanisms.
- Monitoring and Debugging: We’ve highlighted the significance of monitoring queue health, logging errors, and using debugging tools.
- Advanced Techniques: We’ve delved into job middleware, scheduling jobs, and testing strategies.
By following these best practices, you can significantly improve the performance and scalability of your Laravel applications. So, go ahead, embrace the power of background jobs, and take your Laravel projects to new heights!